Enhancing Collaboration: Unlocking the Potential of Slack API
Developers can enhance their Slack workspaces with custom apps and automated workflows using the Slack API—a powerful toolkit for boosting team collaboration and productivity.
The Slack API allows developers to build custom integrations, chatbots, and automated processes that seamlessly interact with Slack’s platform. But what exactly can you do with this versatile API?
The Slack API is a collection of specialized APIs, each designed for specific purposes: the Web API, Events API, and Real-Time Messaging (RTM) API. Here’s a closer look at each:
The Web API serves as the foundation, allowing developers to query information from a Slack workspace or make changes to it. Whether creating new channels, sending messages, or retrieving user data, the Web API is essential.
The Events API enables applications to subscribe to real-time Slack events. For example, you can build a bot that responds instantly to specific message posts or channel changes.
The Real-Time Messaging API is perfect for applications needing instant, two-way communication with Slack, like real-time chatbots or alert systems.
OAuth underpins these APIs, ensuring secure authentication and data privacy for Slack integrations. It allows custom apps to safely access Slack resources.
With the Slack API, you can automate routine tasks, create custom reporting tools, or develop interactive games for team building. The possibilities are nearly endless, limited only by your imagination.
Explore how the Slack API can transform your workspace. In the following sections, we’ll delve deeper into each API, exploring real-world applications and best practices for leveraging Slack’s platform. Unlock a new level of productivity for your team!
Web API Essentials for App Integration
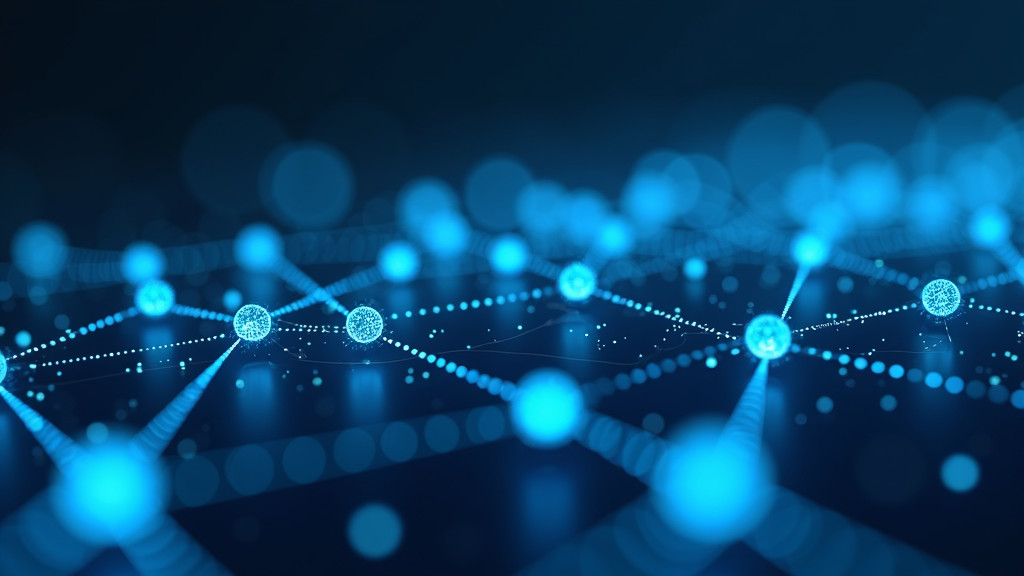
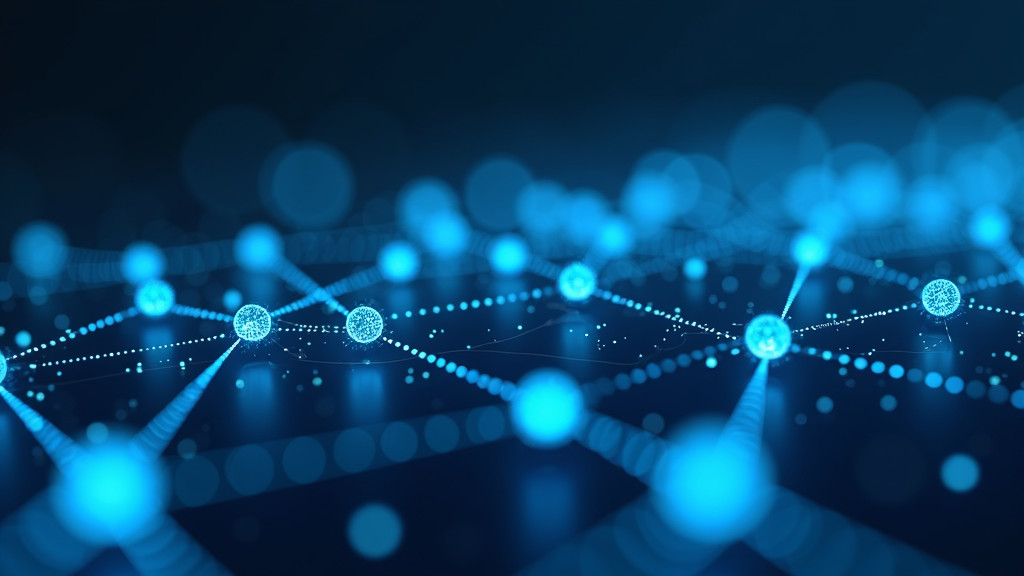
Slack’s Web API is a vital tool for app integrations, providing developers with the resources to create engaging experiences within Slack. The Web API uses HTTP methods to facilitate interaction with Slack’s infrastructure, enabling apps to send messages, modify channels, and access Slack data.
The Web API’s versatility is a major advantage. The chat.postMessage
method, for example, lets apps send messages to channels or users efficiently. Here’s a simple Node.js example:
const result = await client.chat.postMessage({
channel: 'C1234567890',
text: 'Hello, Slack!'
});
This code snippet illustrates how easy it is to send messages programmatically, enabling automated notifications and chatbots.
Channel modifications are another key feature. Methods like conversations.create
and conversations.invite
enable dynamic Slack workspace management. For instance, an onboarding app could create project channels and invite team members automatically:
await client.conversations.create({
name: 'new-project-2024',
is_private: false
});
Authentication is crucial for these features. OAuth tokens unlock the Web API’s capabilities. Without valid tokens, your app can’t perform actions. As Slack’s OAuth documentation explains, “OAuth allows a user in any Slack workspace to install your app.”
Message formatting is another strength of the Web API. With Block Kit, developers can craft visually appealing and interactive messages. From text formatting to interactive elements, the options are broad:
await client.chat.postMessage({
channel: 'C1234567890',
blocks: [
{
type: 'section',
text: {
type: 'mrkdwn',
text: 'Hello, *world*!'
},
accessory: {
type: 'button',
text: {
type: 'plain_text',
text: 'Click Me'
},
action_id: 'button_click'
}
}
]
});
This example demonstrates sending a message with formatted text and an interactive button, highlighting the flexibility of Block Kit components.
Overall, Slack’s Web API is a crucial resource for developers aiming to create advanced app integrations. By using its HTTP methods for messaging, channel management, and data interaction, along with OAuth authentication and rich formatting options, developers can build apps that significantly enhance the Slack experience.
Leveraging the Events API for Real-Time Updates
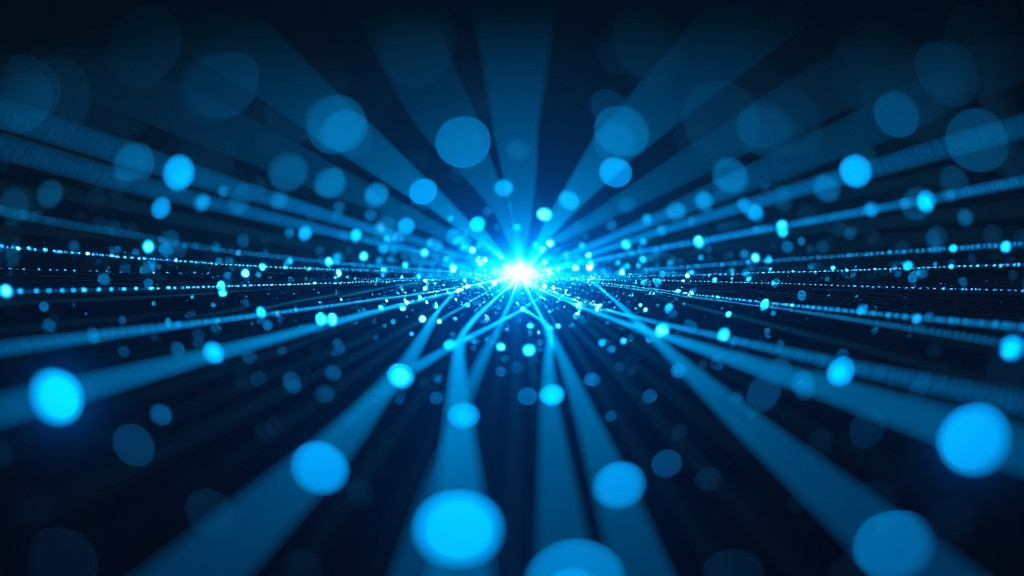
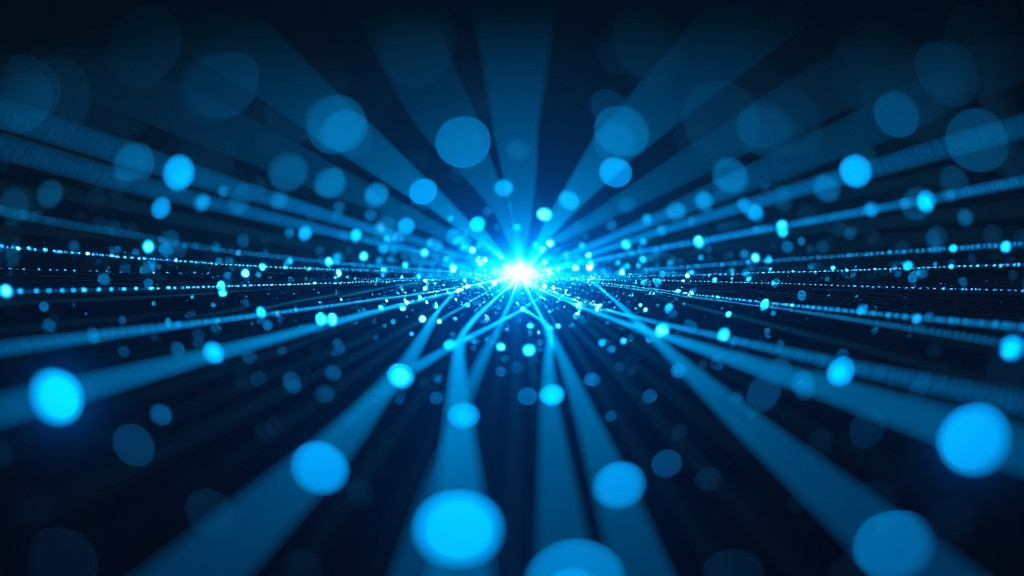
A sleek representation of data convergence in a photorealistic style. – Artist Rendition
Imagine your Slack app instantly responding to every message, reaction, or file upload in your workspace. That’s the power of Slack’s Events API. This tool allows developers to create applications that react in real-time to user actions, making your Slack experience more dynamic and responsive.
The Events API is a streamlined way for apps to listen and respond to activities happening in Slack. It’s like having a vigilant assistant constantly monitoring your workspace, ready to spring into action. Whether it’s a new message in a channel, a file being shared, or a team member joining, your app can be alerted instantly.
So how does this magic happen? It’s all about event subscriptions. Developers can choose specific events they want their app to subscribe to. When one of these events occurs, Slack sends a JSON payload to the app’s designated endpoint. It’s like getting a personalized news feed for your Slack workspace, but instead of articles, you’re getting real-time updates on user actions.
The Power of Real-Time Responsiveness
Imagine you’ve created a project management bot for your team. With the Events API, your bot could:
- Automatically create a new task when a team member posts a message with a specific keyword
- Send a welcome message to new members as soon as they join a channel
- Update project timelines instantly when files are uploaded to specific channels
- Trigger notifications when important messages are reacted to with a particular emoji
The possibilities are endless, limited only by your imagination and coding skills. By leveraging the Events API, you’re not just building a Slack app – you’re creating a responsive, intelligent assistant that can enhance your team’s productivity in real-time.
Use Case | Platform | Description |
---|---|---|
Image Processing Notification | Azure Event Grid | Notifies when an image is uploaded to a storage account. |
Security Alerts | Secret Server | Alerts administrators of configuration changes or security issues. |
Real-Time Equipment Updates | Azure Event Domains | Provides real-time updates on equipment maintenance and system health. |
Streamlined Event Handling with Socket Mode
While the traditional method of handling events involves setting up a public HTTP endpoint, Slack has introduced a more streamlined approach called Socket Mode. This feature allows your app to receive events through a WebSocket connection, eliminating the need for a public-facing server.
Socket Mode is particularly useful for developers working in restricted network environments or those who want to simplify their app’s architecture. It’s like having a direct, always-open phone line to Slack, ready to receive event notifications at any time.
Here’s a simple example of how you might handle an event using Socket Mode in Python:
from slack_bolt.adapter.socket_mode import SocketModeHandler
from slack_bolt import Appapp = App(token=”YOUR_BOT_TOKEN”)
@app.event(“message”)
def handle_message_events(body, logger):
logger.info(body)SocketModeHandler(app, “YOUR_APP_TOKEN”).start()
This code sets up a basic event listener for message events. When a message is posted in a channel your bot has access to, this function will be triggered, allowing you to process and respond to the message in real-time.
The Events API, especially when combined with Socket Mode, offers a powerful, efficient way to create responsive Slack apps. By tapping into the real-time flow of information in your workspace, you can build tools that not only react to user actions but anticipate needs and streamline workflows. It’s not just about building smarter apps – it’s about creating a more connected, efficient Slack experience for your entire team.
Choosing Between RTM and Events API
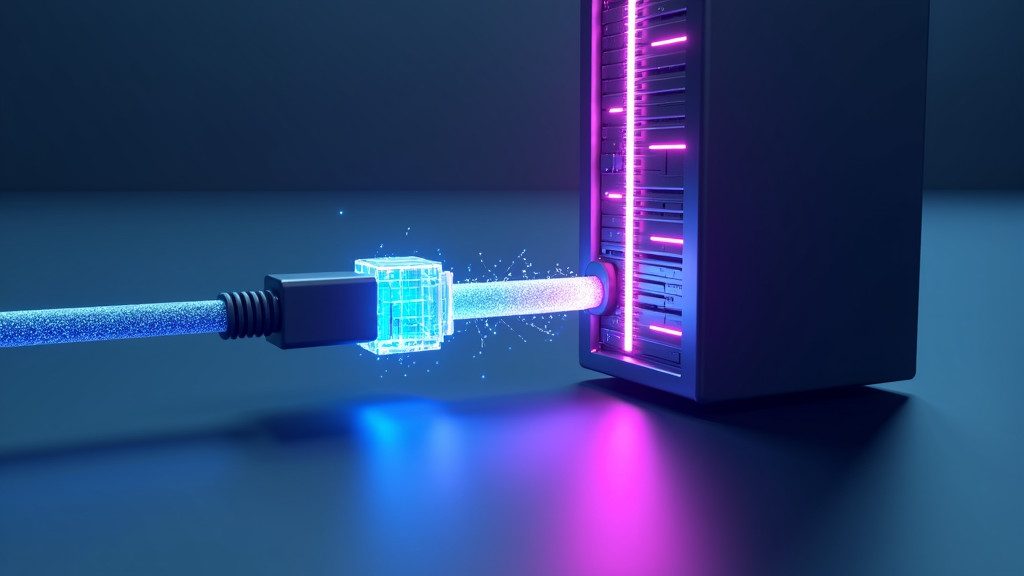
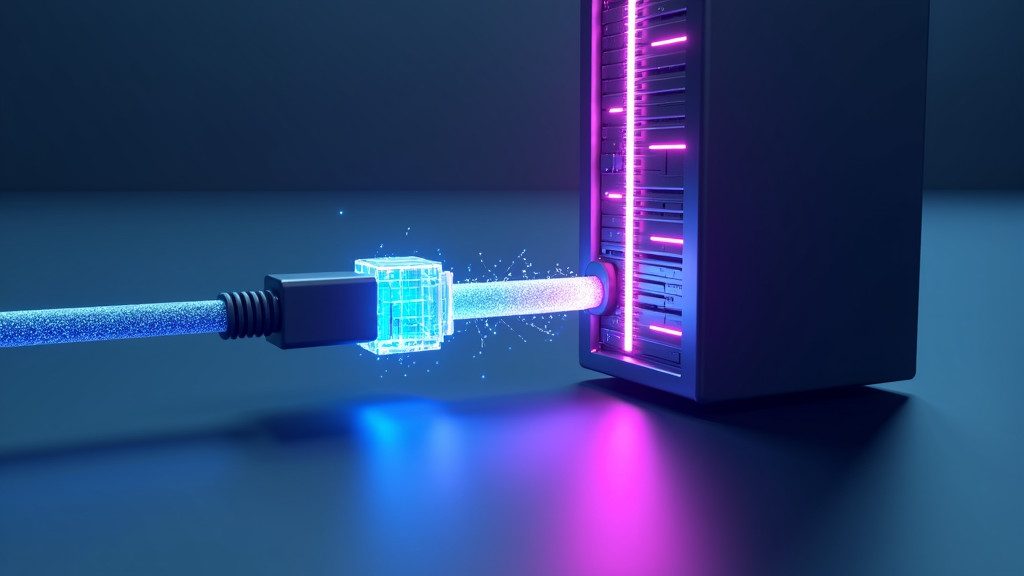
A photorealistic 3D representation of networking technologies, with WebSocket on one side and HTTP requests on the other. – Artist Rendition
Developers often face a key decision when implementing real-time messaging in Slack: choosing between the Real Time Messaging (RTM) API and the Events API. While both serve similar purposes, their use cases differ. Here’s a breakdown of the key differences to guide your decision.
The RTM API, using WebSocket, excels in scenarios requiring instant, bidirectional communication. It suits applications focused on direct messaging or those needing a constant connection to Slack. For instance, if you’re building a chat bot that must respond immediately, RTM is a strong option.
Conversely, the Events API offers flexibility with HTTP POST requests to deliver events, ideal for broad event tracking. This API is perfect for handling a wide range of Slack events beyond messaging, such as channel creations or user status changes.
Decision Flowchart: RTM or Events API?
1. Does your app need to send and receive messages in real-time?
– If YES, consider RTM API
– If NO, proceed to question 2
2. Do you need to track a variety of Slack events beyond messaging?
– If YES, choose Events API
– If NO, proceed to question 3
3. Is your app deployed behind a firewall with limited external access?
– If YES, RTM API might be necessary
– If NO, Events API is generally recommended
Slack is gradually phasing out the RTM API in favor of the Events API, reflecting an industry trend towards scalable, event-driven architectures. The Events API’s subscription model allows your application to receive only necessary events, reducing data transfer and processing, which is crucial as your user base grows or if you’re dealing with large Slack workspaces.
The decision between RTM and Events API depends on your use case. While a simple real-time chat bot might use RTM, most modern Slack applications requiring broader functionality and scalability should opt for the Events API. It offers greater flexibility, better performance at scale, and aligns with Slack’s development direction.
Best Practices for API Authentication and Security
Securing your Slack app’s API authentication is crucial for protecting both your application and its users. Here are some critical best practices for managing OAuth tokens and access permissions effectively.
Implement Secure Token Storage
Storing OAuth tokens securely is your first line of defense against unauthorized access. Avoid storing tokens in plain text or exposing them in client-side code. Use secure storage systems suitable for your platform.
For mobile platforms, use Keystore for Android or Keychain Services for iOS. On server-side applications, leverage secret management services like Google Cloud Secret Manager to safeguard your tokens. Treat tokens with the same caution as passwords: encrypt them at rest and never transmit them over insecure channels.
Employ Token Rotation and Expiration
Enhance security by implementing token rotation, which involves regularly replacing old tokens with new ones, reducing the window of opportunity for potential attackers. Set reasonable expiration times for access tokens.
When refreshing tokens, use the oauth.v2.access
method with the refresh token to obtain a new access token. Always revoke old tokens immediately after refreshing to maintain a clean security posture.
const response = await axios.post(‘https://slack.com/api/oauth.v2.access’, {client_id: CLIENT_ID, client_secret: CLIENT_SECRET, grant_type: ‘refresh_token’, refresh_token: REFRESH_TOKEN});
Slack API Documentation
Utilize Secure Authorization Flows
When implementing OAuth 2.0, always use secure authorization flows. For server-side applications, the Authorization Code flow is recommended as it keeps sensitive credentials server-side, away from potential client-side vulnerabilities. Avoid using the Implicit Grant flow for production applications, as it exposes access tokens to the client side. Redirect users to Slack’s authorization page and handle the token exchange server-side.
Implement Proper Scopes and Permissions
Adhere to the principle of least privilege when requesting scopes for your Slack app. Only request the permissions your app absolutely needs to function. This limits potential damage if a token is compromised. Regularly audit your app’s scopes and remove any unnecessary permissions. Slack provides granular scopes, allowing you to fine-tune your app’s access levels precisely.
Secure Your Redirect URIs
Carefully manage your app’s redirect URIs. Only use HTTPS URLs that you control and avoid using localhost or IP addresses in production environments. Regularly review and update your redirect URIs in the Slack App settings to prevent potential exploitation. A compromised redirect URI can lead to token interception, so treat them as sensitive configuration items.
Implement robust CSRF protection for your redirect endpoints to prevent attacks leveraging your OAuth flow.
Monitor and Respond to Security Events
Implement comprehensive logging and monitoring for your app’s authentication processes. Watch for unusual patterns, such as multiple failed token refreshes or unexpected IP addresses making requests. Consider integrating with Slack’s Cross-Account Protection service to receive real-time notifications about critical security events, including token revocations.
By following these best practices, you’ll significantly enhance the security of your Slack app’s API authentication. Security is an ongoing process, so regularly review and update your security measures to stay ahead of potential threats.
Utilizing the Slack API with SmythOS
SmythOS transforms Slack API integration, providing a powerful platform that simplifies workflow. The drag-and-drop interface eliminates complex coding, enabling even beginners to create advanced AI agents that interact seamlessly with Slack.
A key feature of SmythOS is its visual design option, which accelerates development. Unlike traditional platforms that require coding expertise, SmythOS’s intuitive interface allows users to build intricate Slack integrations quickly and with fewer errors.
The platform’s built-in testing and debugging tools further enhance its appeal. These tools facilitate real-time troubleshooting, helping developers maintain the integrity and efficiency of Slack integrations, especially in dynamic business settings.
SmythOS also excels in business system integration. With access to over 300,000 pre-built integrations, developers can effortlessly connect Slack with numerous business tools and services, making it adaptable to any business environment.
The platform’s flexibility extends to deployment options. SmythOS supports deployment across multiple platforms, including ChatGPT, Discord, and web applications, ensuring businesses can maximize their Slack integrations’ impact.
Key Advantages of SmythOS for Slack API Integration
SmythOS offers several advantages over traditional methods. Its no-code approach democratizes development, allowing teams to collaborate effectively, regardless of technical expertise, fostering innovation and faster project completion.
The visual workflow creator is another significant advantage, enabling developers to design complex Slack API interactions without coding syntax. This visual method speeds up development and simplifies conceptualizing and refining integration logic.
SmythOS’s comprehensive integration capabilities set it apart. Connecting with over 200 million APIs ensures developers can create Slack integrations that interact with any external service or data source.
SmythOS streamlines AI integration across industries, offering scalable solutions for enhancing operational efficiency and driving innovation.
Feature | SmythOS | Traditional Platforms |
Development Approach | No-code, drag-and-drop interface | Code-intensive, requires technical expertise |
Integration Options | 300,000+ integrations | Limited integrations |
Deployment Capabilities | Universal, including ChatGPT, Slack, and web apps | Platform-specific |
Target Audience | Developers and non-developers | Primarily developers |
SmythOS represents a leap in Slack API integration technology. Its visual design tools, extensive integration options, and debugging features make it invaluable for developers creating sophisticated Slack integrations. As businesses rely on Slack for communication, SmythOS is set to shape the future of workplace productivity tools.
Effective Strategies for Error Handling in Slack Apps
Developing a Slack app that runs smoothly requires robust error handling. Without it, your app may crash unexpectedly or fail to respond properly, frustrating users and potentially damaging your app’s reputation. Here are some common error types Slack developers encounter and strategies to manage them effectively.
Understanding API Rate Limits
API rate limits are a common issue for Slack app developers. Slack imposes these limits to ensure fair usage and prevent server overload. When your app exceeds these limits, it receives a 429 ‘Too Many Requests’ error.
To handle rate limits gracefully, implement exponential backoff. This strategy involves waiting for progressively longer periods before retrying a failed request. Here’s a simple example:
if (response.status === 429) { const retryAfter = response.headers.get(‘Retry-After’); await delay(retryAfter * 1000); return makeRequest(); // Retry the request }
Adapted from Slack’s Rate Limits documentation
By respecting these limits, you ensure your app remains responsive and doesn’t get temporarily blocked from making API calls.
Tackling Authentication Failures
Authentication errors can occur when tokens expire or become invalid, typically manifesting as 401 ‘Unauthorized’ errors. To mitigate this, implement a token refresh mechanism and proper error handling for authentication failures.
If you encounter a 401 error, prompt the user to re-authenticate or automatically refresh the token if possible. Always store tokens securely and never expose them in client-side code or log files.
Handling Network Issues
Network connectivity problems can cause requests to fail or time out. Implement retry logic with a maximum number of attempts to handle temporary network glitches. For example:
async function makeRequestWithRetry(maxAttempts = 3) { for (let attempt = 1; attempt <= maxAttempts; attempt++) { try { return await makeRequest(); } catch (error) { if (attempt === maxAttempts) throw error; await delay(1000 * attempt); // Wait longer between each retry } } }
This approach gives your app a better chance of succeeding when network conditions are unreliable.
Dealing with Unexpected Errors
For unforeseen errors, implement a global error handler. This catch-all solution ensures that any unhandled exceptions are logged and don’t crash your app. Consider sending these logs to a monitoring service for easier debugging.
Provide user-friendly error messages. Instead of exposing technical details, offer clear instructions on what users can do next, such as retrying the action or contacting support.
Monitoring and Logging
Proactive error management involves monitoring your app’s performance and logging errors for analysis. Use Slack’s Audit Logs API to track important events and identify patterns that might indicate recurring issues.
Implement structured logging to capture contextual information with each error. This practice makes it easier to reproduce and fix issues quickly.
Testing Error Scenarios
Don’t wait for errors to occur in production. Simulate various error conditions during development and testing phases. Create unit tests that cover different error scenarios and edge cases to ensure your error handling code works as expected.
By implementing these strategies, you’ll create a more resilient Slack app that can handle errors gracefully, providing a better experience for your users and making your life as a developer much easier. Effective error handling is not just about preventing crashes; it’s about creating a robust, user-friendly application that can withstand the unexpected.
Conclusion and Final Thoughts
Integrating Slack APIs can significantly enhance digital assistants, improving communication and productivity. The key is for developers to manage API functionalities while ensuring user data security.
SmythOS offers tools to tackle these challenges, focusing on pre-built API integrations. This platform simplifies development, allowing teams to innovate without common hurdles. The synergy between Slack’s API ecosystem and SmythOS’s tools fosters rapid development of advanced digital assistants.
With AI and machine learning advancing, the future of Slack API integration looks promising. These technologies will offer more intuitive and secure ways to utilize Slack in digital workflows. For developers, adopting platforms like SmythOS could unlock new efficiency and creativity levels in their projects.
API integration is an ongoing journey, presenting new opportunities and challenges. By using the right tools and prioritizing security and innovation, developers can ensure their digital assistants surpass modern workspace needs. With Slack APIs as a foundation and SmythOS as a catalyst, the potential for transformative digital experiences is vast.
Last updated:
Disclaimer: The information presented in this article is for general informational purposes only and is provided as is. While we strive to keep the content up-to-date and accurate, we make no representations or warranties of any kind, express or implied, about the completeness, accuracy, reliability, suitability, or availability of the information contained in this article.
Any reliance you place on such information is strictly at your own risk. We reserve the right to make additions, deletions, or modifications to the contents of this article at any time without prior notice.
In no event will we be liable for any loss or damage including without limitation, indirect or consequential loss or damage, or any loss or damage whatsoever arising from loss of data, profits, or any other loss not specified herein arising out of, or in connection with, the use of this article.
Despite our best efforts, this article may contain oversights, errors, or omissions. If you notice any inaccuracies or have concerns about the content, please report them through our content feedback form. Your input helps us maintain the quality and reliability of our information.