Reinforcement Learning in TensorFlow: A Comprehensive Guide
Imagine a world where machines learn from their mistakes, continuously improving their performance to achieve complex goals. This is the fascinating realm of reinforcement learning (RL) in TensorFlow. This advanced approach to machine learning is transforming how we build intelligent systems, from game-playing AIs to robotic control.
At its core, RL is about teaching agents to make decisions in dynamic environments. Unlike traditional supervised learning, RL agents learn through trial and error, similar to humans. They take actions, observe outcomes, and adjust their strategies to maximize long-term rewards. This adaptability makes RL powerful and applicable to various real-world problems.
TensorFlow, Google’s open-source machine learning platform, has become a go-to tool for implementing RL algorithms. Its TensorFlow Agents (TF-Agents) library provides a robust framework for developing and deploying RL models, making it accessible to both seasoned researchers and ambitious developers.
This article will explore reinforcement learning in TensorFlow. We’ll unpack the fundamental concepts driving RL, explore the powerful features of TF-Agents, and engage in practical implementations. Whether you’re new to the field or a seasoned data scientist, prepare to delve into the future of intelligent decision-making.
Main Takeaways:
- Reinforcement learning enables agents to learn optimal behaviors through interaction with their environment
- TensorFlow and its TF-Agents library provide a comprehensive toolkit for implementing RL algorithms
- We’ll cover RL fundamentals, TF-Agents features, and practical implementation techniques
Fundamentals of Reinforcement Learning
Reinforcement learning (RL) is a powerful approach in artificial intelligence where an agent learns to make optimal decisions by interacting with its environment. Unlike other machine learning methods, RL doesn’t rely on labeled data. Instead, it uses a trial-and-error process to discover the best actions to take in different situations.
At its core, RL involves five key components: the agent, environment, actions, states, and rewards. Let’s break these down in simple terms:
The Agent and Environment
The agent is the learner or decision-maker in RL. It could be a robot, a computer program, or any entity capable of taking actions. The environment is everything the agent interacts with – it’s the world in which the agent operates.
Imagine a robot (the agent) learning to navigate a maze (the environment). The robot must figure out how to reach the exit by trying different paths and learning from its experiences.
Actions and States
Actions are the choices an agent can make. In our maze example, the robot’s actions might include moving forward, turning left, or turning right. The state represents the current situation of the agent within the environment. For the robot, this could be its position in the maze and what it ‘sees’ around it.
Environment | Agent Actions | Environment States |
---|---|---|
Asteroids (Atari game) | Shoot laser, move ship | Positions of asteroids, ship position, score, lives left |
Maze Navigation | Move forward, turn left, turn right | Robot’s position in the maze, visible walls |
CartPole | Move cart left, move cart right | Position and velocity of cart, angle and angular velocity of pole |
Shower Temperature Control | Increase temperature, decrease temperature | Current temperature of the shower |
Each time the agent takes an action, it transitions from one state to another. This process of taking actions and moving between states is at the heart of RL.
Rewards and Learning
The reward is the feedback the agent receives after taking an action. It’s how the agent learns which actions are good and which are bad. In our maze scenario, reaching the exit might give a large positive reward, while hitting a wall could result in a small negative reward.
Over time, the agent develops a policy – a strategy for choosing actions in different states. The goal is to maximize the cumulative reward over time, not just immediate rewards. This is why RL is so powerful for solving complex, long-term problems.
RL is based on the principle that all goals can be described by maximizing expected cumulative reward.
The Learning Process
The RL process is cyclical. The agent observes the current state, chooses an action based on its policy, receives a reward, and transitions to a new state. This cycle repeats, with the agent continuously updating its policy to make better decisions.
One of the key challenges in RL is balancing exploration (trying new actions to gather more information) with exploitation (using known good actions to maximize reward). An effective RL agent must strike a balance between these two strategies.
As the agent interacts with its environment more, it gradually improves its policy. This improvement comes from updating the agent’s understanding of the value of different state-action pairs – essentially, how good it is to take a particular action in a given state.
RL has found applications in various fields, from game-playing AI that can beat human champions to robotics and autonomous vehicles. Its ability to learn complex behaviors without explicit programming makes it a powerful tool in the AI toolkit.
Understanding these fundamentals of reinforcement learning provides a solid foundation for exploring more advanced concepts and applications in this exciting field of artificial intelligence.
Introduction to TensorFlow Agents
Reinforcement learning (RL) stands out as a powerful approach for training intelligent agents. TensorFlow Agents (TF-Agents) offers a robust toolkit for researchers and developers alike.
TF-Agents is an open-source library built on TensorFlow that streamlines the process of developing and testing RL algorithms. It aims to make the implementation of cutting-edge RL techniques more accessible and efficient.
At its core, TF-Agents provides tools for environment interaction, policy optimization, and algorithm implementation. This modular architecture allows for rapid experimentation and iterative development of RL models.
Key Features of TF-Agents
TF-Agents includes pre-implemented algorithms like DQN, PPO, and DDPG, saving developers countless hours of coding and debugging.
The library also offers flexible environment wrappers, allowing seamless integration with various simulation frameworks. This versatility enables researchers to focus on algorithm design rather than environment setup.
TF-Agents excels in policy optimization. It provides utilities for defining and training policies, including neural network architectures specifically designed for RL tasks.
TF-Agents makes designing, implementing and testing new RL algorithms easier by providing well-tested modular components that can be modified and extended.
TensorFlow.org
Another crucial aspect of TF-Agents is its robust suite of utilities for data collection and replay buffers. These components are essential for efficient training of RL agents, especially in complex environments.
Building and Training RL Models
TF-Agents simplifies the process of building and training RL models through its intuitive API. Developers can define environments, policies, and agents with just a few lines of code, as demonstrated in this example:
Component | Description | Code Example |
---|---|---|
Environment Setup | Load the CartPole environment for training and evaluation | import tensorflow as tf from tf_agents.environments import suite_gym train_env = suite_gym.load(‘CartPole-v0’) eval_env = suite_gym.load(‘CartPole-v0’) |
Q-Network Definition | Create a neural network to predict the value of taking each action in a given state | from tf_agents.networks import q_network q_net = q_network.QNetwork( train_env.observation_spec(), train_env.action_spec(), fc_layer_params=(100,) ) |
DQN Agent Creation | Instantiate the DQN agent with the Q-network, optimizer, and loss function | from tf_agents.agents.dqn import dqn_agent agent = dqn_agent.DqnAgent( train_env.time_step_spec(), train_env.action_spec(), q_network=q_net, optimizer=tf.compat.v1.train.AdamOptimizer(learning_rate=1e-3), td_errors_loss_fn=tf.compat.v1.losses.mean_squared_error ) |
Replay Buffer Setup | Create a buffer to store past experiences for the agent to learn from | from tf_agents.replay_buffers import tf_uniform_replay_buffer replay_buffer = tf_uniform_replay_buffer.TFUniformReplayBuffer( data_spec=agent.collect_data_spec, batch_size=train_env.batch_size, max_length=100000 ) |
Training Loop | Collect experiences, train the agent, and periodically evaluate its performance | # Collect initial experiences initial_collect_driver.run() # Training loop for _ in range(num_iterations): # Collect experience collect_driver.run() # Sample a batch of data from the buffer and update the agent’s network experience, _ = next(iterator) train_loss = agent.train(experience) # Periodically evaluate the agent’s performance if step % eval_interval == 0: avg_return = compute_avg_return(eval_env, agent.policy, num_eval_episodes) print(f’Step = {step}: Average Return = {avg_return}’) |
Performance Evaluation | Plot the average return to see how the agent improves over time | import matplotlib.pyplot as plt plt.plot(returns) plt.title(‘Average Return vs Training Steps’) plt.xlabel(‘Training Steps’) plt.ylabel(‘Average Return’) plt.show() |
TF-Agents’ documentation provides comprehensive guides and tutorials, making it easier for newcomers to get started with RL development.
The library’s integration with TensorFlow’s ecosystem offers additional benefits, such as easy model deployment and compatibility with TensorFlow’s visualization tools like TensorBoard.
By leveraging TF-Agents, researchers and developers can accelerate their RL projects, from concept to deployment, while maintaining the flexibility to implement custom algorithms when needed.
Implementing Reinforcement Learning with TF-Agents
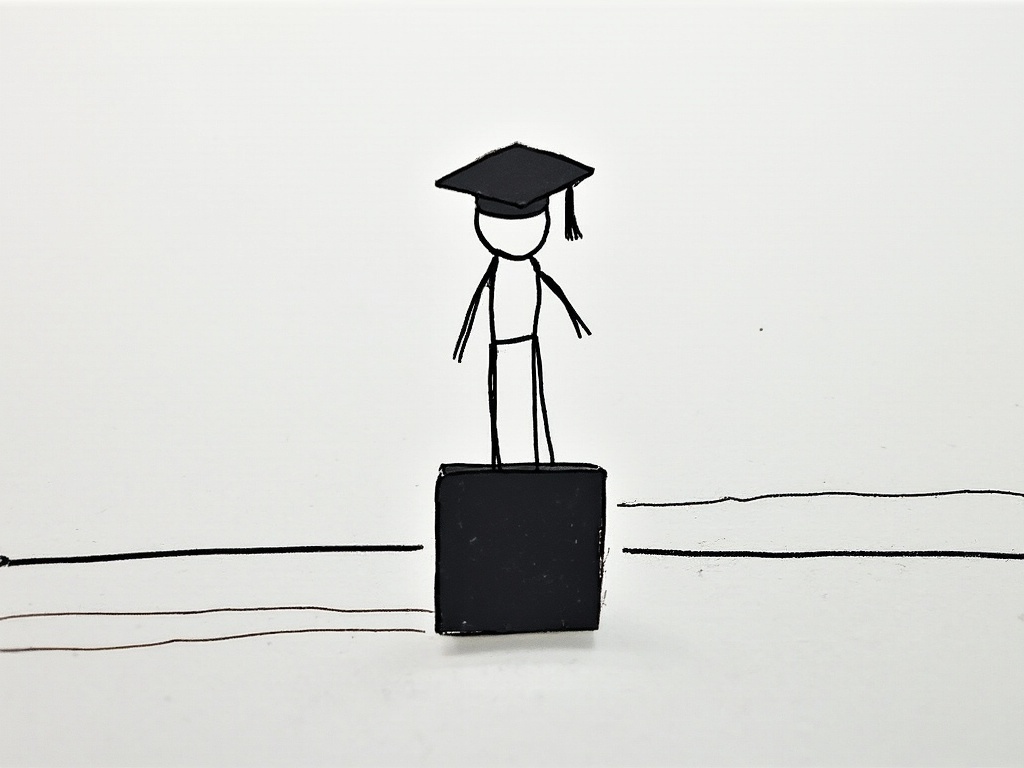
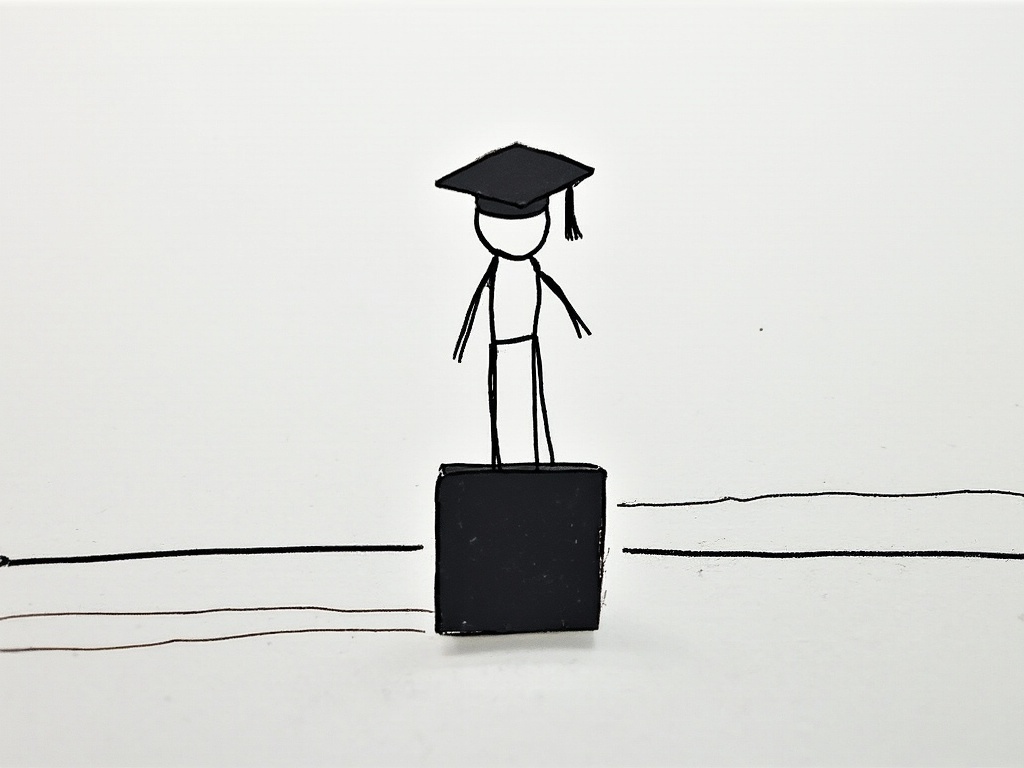
Reinforcement learning (RL) is a powerful approach for teaching agents to make decisions in complex environments. This section covers the process of implementing a Deep Q Network (DQN) agent using TF-Agents, a flexible library for RL in TensorFlow. We’ll use the classic CartPole environment where the goal is to balance a pole on a moving cart.
Setting Up the Environment
The first step is to set up the CartPole environment. TF-Agents makes this process straightforward:
import tensorflow as tf
from tf_agents.environments import suite_gym
train_env = suite_gym.load('CartPole-v0')
eval_env = suite_gym.load('CartPole-v0')
These environments will be used for training and evaluation. The CartPole environment provides a simple yet challenging task: keep a pole balanced on a cart by moving the cart left or right.
Defining the Q-Network
At the heart of our DQN agent is the Q-network, which predicts the value of taking each action in a given state. We’ll create a simple neural network using TF-Agents’ QNetwork class:
from tf_agents.networks import q_network
q_net = q_network.QNetwork(
train_env.observation_spec(),
train_env.action_spec(),
fc_layer_params=(100,)
)
This network has a single hidden layer with 100 neurons. It takes the environment’s state as input and outputs a value for each possible action.
Creating the DQN Agent
Now that we have our Q-network, we can create the DQN agent:
from tf_agents.agents.dqn import dqn_agent
agent = dqn_agent.DqnAgent(
train_env.time_step_spec(),
train_env.action_spec(),
q_network=q_net,
optimizer=tf.compat.v1.train.AdamOptimizer(learning_rate=1e-3),
td_errors_loss_fn=tf.compat.v1.losses.mean_squared_error
)
The agent uses the Adam optimizer and mean squared error for its loss function. These choices work well for many RL tasks, but feel free to experiment with different options.
Setting Up the Replay Buffer
A crucial component of DQN is the replay buffer, which stores past experiences for the agent to learn from:
from tf_agents.replay_buffers import tf_uniform_replay_buffer
replay_buffer = tf_uniform_replay_buffer.TFUniformReplayBuffer(
data_spec=agent.collect_data_spec,
batch_size=train_env.batch_size,
max_length=100000
)
This buffer can hold up to 100,000 experiences, allowing our agent to learn from a diverse set of past interactions.
Training the Agent
With all components in place, we can now train our agent. The training loop involves collecting experiences and using them to update the agent’s Q-network:
# Collect initial experiences
initial_collect_driver.run()
# Training loop
for _ in range(num_iterations):
# Collect experience
collect_driver.run()
# Sample a batch of data from the buffer and update the agent's network
experience, _ = next(iterator)
train_loss = agent.train(experience)
# Periodically evaluate the agent's performance
if step % eval_interval == 0:
avg_return = compute_avg_return(eval_env, agent.policy, num_eval_episodes)
print(f'Step = {step}: Average Return = {avg_return}')
This loop collects experiences, trains the agent, and periodically evaluates its performance. The compute_avg_return
function (not shown) runs the agent’s policy in the evaluation environment to measure its effectiveness.
Evaluating Performance
To see how our agent improves over time, we can plot its average return:
import matplotlib.pyplot as plt
plt.plot(returns)
plt.title('Average Return vs Training Steps')
plt.xlabel('Training Steps')
plt.ylabel('Average Return')
plt.show()
As training progresses, you should see the average return increase, indicating that the agent is learning to balance the pole for longer periods.
By following these steps, you’ve implemented a DQN agent using TF-Agents and trained it on the CartPole environment. This process can be adapted to other environments and RL algorithms, opening up a world of possibilities for training intelligent agents. Remember, RL is often a process of trial and error, so don’t be discouraged if your agent doesn’t perform perfectly right away. Experiment with different hyperparameters, network architectures, and even alternative algorithms to find what works best for your specific problem.
Advanced Features of TF-Agents
TF-Agents goes beyond basic reinforcement learning algorithms, offering a suite of advanced features that elevate its capabilities for complex real-world applications. Here are some of these cutting-edge functionalities that make TF-Agents a powerhouse for researchers and practitioners alike.
Multi-Armed Bandits: Balancing Exploration and Exploitation
At the forefront of TF-Agents’ advanced toolkit are multi-armed bandits, a class of algorithms designed to tackle the exploration-exploitation dilemma. Imagine a scenario where a recommendation system must choose between different product options to maximize user engagement.
Multi-armed bandits in TF-Agents provide a framework for making these decisions efficiently. They allow the agent to learn from past interactions and gradually refine its strategy, balancing the need to explore new options with exploiting known high-performing choices.
For instance, an e-commerce platform could use multi-armed bandits to optimize its homepage layout. Each ‘arm’ of the bandit represents a different design, and the algorithm learns over time which layouts lead to higher conversion rates.
Multi-armed bandits shine in scenarios where quick adaptation is crucial, and the cost of exploration is relatively low.
Riquelme et al., Deep Bayesian Bandits Showdown (2018)
Contextual Bandits: Adding Nuance to Decision-Making
Taking the concept a step further, TF-Agents implements contextual bandits, which consider additional information about the environment or user when making decisions. This added context allows for more nuanced and personalized strategies.
A practical application of contextual bandits can be found in personalized news recommendation systems. Here, the ‘context’ might include factors like the user’s reading history, time of day, or current events. The bandit algorithm then uses this context to select articles that are most likely to interest the specific user.
TF-Agents’ tutorial on contextual bandits provides a hands-on example using the ‘Mushroom Environment’, where an agent learns to distinguish between edible and poisonous mushrooms based on their features.
Customizable Training Loops: Flexibility for Researchers
For those pushing the boundaries of RL research, TF-Agents offers customizable training loops. This feature allows researchers and advanced practitioners to have granular control over the learning process, enabling the implementation of novel algorithms or the fine-tuning of existing ones.
With customizable training loops, you can modify how agents interact with environments, adjust reward calculations, or implement custom exploration strategies. This level of flexibility is invaluable for tackling unique problems or optimizing performance in specific domains.
For example, in a robotic control task, a researcher might use customizable training loops to implement a curriculum learning approach. The difficulty of the task could be gradually increased as the agent improves, leading to more robust and generalizable policies.
Practical Impact of Advanced Features
These advanced features significantly enhance the flexibility and scalability of reinforcement learning models built with TF-Agents. Multi-armed and contextual bandits allow for efficient learning in scenarios with limited feedback, while customizable training loops open the door to innovative approaches in complex environments.
From optimizing ad placements to developing adaptive traffic light systems, the applications of these advanced TF-Agents features are vast and impactful. As the field of reinforcement learning continues to evolve, TF-Agents stands ready to support cutting-edge research and real-world implementations alike.
Examples of practical applications of advanced TF-Agents features
Benefits of Using SmythOS for Reinforcement Learning
SmythOS stands out as a game-changer in reinforcement learning (RL), offering features that streamline development and enhance reliability. Its sophisticated visual builder empowers developers to construct complex RL agents through an intuitive drag-and-drop interface.
One standout feature is its advanced visual debugging capabilities. This tool provides real-time insights into RL agent performance, allowing developers to track key metrics, identify bottlenecks, and optimize models with ease. By offering a clear window into the inner workings of RL systems, SmythOS enables teams to make data-driven decisions and refine algorithms more effectively.
Enterprise-grade security is another cornerstone of SmythOS, making it an ideal choice for organizations handling sensitive data. The platform implements robust security measures to protect valuable knowledge bases and ensure compliance with data protection regulations, addressing a critical concern for many businesses venturing into RL.
SmythOS’s security features go beyond basic protection, offering a comprehensive suite of tools designed to safeguard RL projects at every stage of development. This level of security is particularly crucial for complex data relationships often encountered in RL applications.
Integration capabilities set SmythOS apart in the RL development ecosystem. The platform offers seamless connection with major graph databases, allowing organizations to leverage their existing data infrastructure while harnessing the power of RL. This integration is valuable for projects dealing with complex, interconnected data structures—a common scenario in enterprise-level applications.
Feature | Description |
---|---|
Universal Integration | Unifies all tools, data, and processes into a single digital ecosystem, streamlining workflow and enhancing analytics and automation. |
AI Collaboration | Enables employees to work alongside AI agents naturally, blending human creativity with AI’s speed and precision. |
Predictive Intelligence | Predicts market trends and internal needs, aiding in decision-making such as inventory adjustments and staffing needs. |
Adaptive Learning | Evolves with the business, continuously optimizing operations and ensuring the tools remain responsive. |
Democratized Innovation | Empowers every employee to become an AI-supported problem solver, unlocking creativity and turning ideas into actionable plans. |
By providing a unified platform that addresses the entire RL development lifecycle, from agent creation to deployment and monitoring, SmythOS significantly reduces the barriers to entry for organizations looking to leverage the power of reinforcement learning. Its combination of visual tools, debugging capabilities, and enterprise features positions it as a transformative force in RL development.
SmythOS isn’t just another AI tool. It’s transforming how we approach AI debugging. The future of AI development is here, and it’s visual, intuitive, and incredibly powerful.Alexander De Ridder, Co-Founder and CTO of SmythOS
For teams handling complex data relationships, SmythOS offers an unparalleled resource. Its built-in tools simplify the development process, allowing developers to focus on creating innovative RL solutions rather than getting bogged down in technical complexities. This efficiency boost can lead to faster development cycles and more robust RL applications.
As reinforcement learning continues to gain traction across industries, tools like SmythOS are becoming indispensable. The platform’s ability to simplify complex processes, integrate with existing infrastructure, and provide robust security makes it an excellent choice for businesses aiming to harness the full potential of RL in their quest for technological advancement.
Conclusion and Future Directions
Reinforcement learning with TensorFlow presents both exciting opportunities and significant challenges. By addressing these hurdles head-on, researchers and developers are paving the way for more robust and effective RL solutions. The future of reinforcement learning looks bright, with ongoing advancements in algorithms and tools promising to push the boundaries of what’s possible.
One key trend to watch is the increasing focus on sample efficiency. As recent developments suggest, researchers are finding innovative ways to train RL agents more effectively with less data. This could dramatically expand the real-world applications of reinforcement learning, especially in domains where large-scale data collection is impractical or costly.
Another area ripe for innovation is the integration of RL with other AI techniques. Hybrid approaches combining reinforcement learning with deep learning or evolutionary algorithms show promise in tackling complex, multi-faceted problems. These synergies could lead to breakthroughs in fields as diverse as robotics, game theory, and autonomous systems.
As the field evolves, platforms like SmythOS are poised to play a crucial role. By offering integrated tools and support for complex RL applications, SmythOS empowers developers to focus on pushing the boundaries of what’s possible rather than getting bogged down in implementation details. Its visual builder and debugging capabilities make it easier than ever to experiment with cutting-edge RL techniques.
Looking ahead, the convergence of advanced RL algorithms, powerful hardware, and intuitive development platforms like SmythOS promises to unlock new frontiers in artificial intelligence. From more sophisticated game-playing agents to adaptive industrial control systems, the potential applications are vast and varied. As we continue to refine these technologies, we’re not just improving algorithms – we’re reshaping how we approach complex decision-making problems across countless domains.
Last updated:
Disclaimer: The information presented in this article is for general informational purposes only and is provided as is. While we strive to keep the content up-to-date and accurate, we make no representations or warranties of any kind, express or implied, about the completeness, accuracy, reliability, suitability, or availability of the information contained in this article.
Any reliance you place on such information is strictly at your own risk. We reserve the right to make additions, deletions, or modifications to the contents of this article at any time without prior notice.
In no event will we be liable for any loss or damage including without limitation, indirect or consequential loss or damage, or any loss or damage whatsoever arising from loss of data, profits, or any other loss not specified herein arising out of, or in connection with, the use of this article.
Despite our best efforts, this article may contain oversights, errors, or omissions. If you notice any inaccuracies or have concerns about the content, please report them through our content feedback form. Your input helps us maintain the quality and reliability of our information.