Programming AI Agents: Unlocking the Future of Intelligent Automation
Programming AI agents involves creating digital entities that can think and act independently. These autonomous entities are designed to perceive their surroundings, make decisions, and take actions to accomplish specific goals. Whether you’re a seasoned developer or a technical leader, understanding how to build and implement AI agents is increasingly crucial in today’s tech landscape.
But what exactly are AI agents, and why should you care about them? AI agents are software programs that can operate independently to solve problems or perform tasks. They power chatbots, autonomous vehicles, and complex systems that manage supply chains or predict market trends.
In this article, we’ll explore:
- The different types of AI agents and how they work
- Step-by-step guides on building your own AI agents
- Popular platforms and tools for agent development
- Tips and tricks for debugging and optimizing your AI creations
Whether you’re looking to streamline your business processes, create innovative applications, or stay ahead in AI technology, understanding AI agents is essential. Let’s get started on this exciting journey into the world of programming AI agents!
What Are AI Agents?
AI agents are poised to revolutionize how we interact with technology. These autonomous digital entities navigate complex environments, make decisions, and carry out tasks without constant human oversight. But what sets them apart from chatbots and virtual assistants?
At their core, AI agents are software programs that perceive their surroundings, process information, and take action to achieve specific goals. They’re proactive problem-solvers that can adapt to new situations. Imagine a digital assistant that doesn’t just tell you the weather but plans your entire day around it, adjusting your schedule and making recommendations based on changing conditions.
AI agents come in various forms, from simple reactive agents to sophisticated learning systems. The simplest agents operate on a basic if-then principle, reacting to stimuli in predefined ways. For example, a thermostat that turns on the heat when the temperature drops below a certain point is a rudimentary form of an AI agent. At the other end of the spectrum are complex agents that can learn from experiences, plan for the future, and even collaborate with other agents or humans.
One of the most exciting aspects of AI agents is their potential to operate in both digital and physical realms. In the digital world, they might manage your email inbox, automatically categorizing messages, drafting responses, and scheduling follow-ups. In the physical world, embodied AI agents could control robots in manufacturing plants or autonomous vehicles navigating city streets.
The grand vision for AI agents is a system that can execute a vast range of tasks, much like a human assistant.
Jim Fan, Senior Research Scientist at Nvidia
However, we’re still in the early stages of AI agent development. Current AI agents face limitations. They can make mistakes, lose track of context in long interactions, or struggle with tasks that require nuanced reasoning. As Kanjun Qiu, CEO of AI startup Imbue, points out, we’re nowhere close to having an agent that can just automate all of these chores for us.
Despite these challenges, the field is advancing rapidly. Tech giants and startups alike are investing heavily in AI agent research, driven by the vision of creating more capable, reliable, and versatile digital assistants. As these technologies evolve, they promise to transform industries, streamline workflows, and perhaps fundamentally alter how we interact with computers and the digital world at large.
Types of AI Agents: From Simple Reactions to Complex Decision Making
Artificial intelligence agents come in various forms, each designed to tackle different levels of complexity in decision-making and environmental interaction. Understanding these types is crucial for anyone looking to harness the power of AI in real-world applications. Let’s explore the main categories of AI agents and how they function.
Reactive Agents: The Simplest Form
At the most basic level, we have reactive agents. These are the AI equivalent of reflexes – they respond directly to their environment without maintaining any internal state or considering past experiences. Imagine a robotic vacuum that simply changes direction when it bumps into a wall. While limited, reactive agents can be incredibly efficient for straightforward tasks.
A classic example of a reactive agent is the thermostat in your home. It doesn’t need to ‘remember’ past temperatures or predict future changes. It simply reacts to the current temperature, turning the heating on or off as needed.
Model-Based Agents: Adding Internal Representation
Moving up in complexity, model-based agents maintain an internal representation of their environment. This ‘model’ allows them to track aspects of the world that may not be immediately observable. These agents can make more informed decisions by considering how their actions might affect their surroundings.
Consider an autonomous car navigating through traffic. It needs to maintain a model of the positions and likely movements of other vehicles, even when they’re temporarily out of sight. This internal representation helps the car make safer decisions about lane changes and turns.
Goal-Based Agents: Striving for Objectives
Goal-based agents take decision-making a step further by working towards specific objectives. These agents don’t just react or model their environment – they actively plan sequences of actions to achieve desired outcomes. This type of agent is particularly useful in complex environments where multiple steps are required to reach a goal.
A prime example of a goal-based agent is a chess-playing AI. It doesn’t just react to the opponent’s last move or model the current board state. Instead, it plans several moves ahead, always working towards the ultimate goal of checkmating the opponent’s king.
Utility-Based Agents: Optimizing for Best Outcomes
At the most sophisticated level, we have utility-based agents. These AI systems not only work towards goals but also evaluate the desirability of different outcomes. They use a utility function to assign value to various states of the world, allowing them to make optimal decisions in complex, multi-objective scenarios.
An advanced trading algorithm is a perfect illustration of a utility-based agent. It doesn’t just aim to make profitable trades (a simple goal) but weighs multiple factors like risk, market volatility, and long-term portfolio balance to maximize overall financial utility.
As we progress from reactive to utility-based agents, we see an increasing capacity for handling complex environments and making nuanced decisions. However, this also comes with greater computational requirements and design complexity. The choice of agent type depends on the specific needs of the application, balancing simplicity with sophistication to achieve optimal results.
Understanding these different types of AI agents is not just academic – it’s crucial for designing effective AI systems in the real world. Whether you’re developing a simple automated response system or a complex decision-making AI for critical applications, knowing which type of agent best suits your needs is the first step towards successful implementation.
Building AI Agents from Scratch
Developing an AI agent from the ground up requires careful planning and execution. At its core, building an AI agent involves crafting a system that can perceive its environment, process information, make decisions, and take actions. Let’s explore the key steps and tools needed to bring an AI agent to life.
Foundations: Choosing Your Tools
Before coding, it’s crucial to select the right programming language and development tools. Python is the de facto language for AI development due to its simplicity, extensive libraries, and robust community support. Here’s why Python stands out:
- Rich ecosystem of AI and machine learning libraries (TensorFlow, PyTorch, scikit-learn)
- Excellent for rapid prototyping and iterative development
- Strong support for data manipulation and analysis
- Integration capabilities with other languages and systems
Alongside Python, you’ll need a solid Integrated Development Environment (IDE). Popular choices include PyCharm, Visual Studio Code, and Jupyter Notebooks, each offering unique features for AI development.
Step-by-Step Guide: Setting Up a Basic AI Agent
Let’s walk through the process of creating a simple AI agent using Python. This agent will demonstrate basic perception and decision-making capabilities.
Environment Setup:
First, ensure you have Python installed on your system. Then, set up a virtual environment to manage dependencies:
python -m venv ai_agent_env source ai_agent_env/bin/activate # On Windows use: ai_agent_env\Scripts\activate
Install Required Libraries:
We’ll use numpy for numerical computations and gym for creating a simple environment:
pip install numpy gym
Create the Agent Class:
Now, let’s define our AI agent with basic perception and decision-making capabilities:
import numpy as np import gym class SimpleAgent: def __init__(self, action_space): self.action_space = action_space def perceive(self, observation): self.current_state = observation def decide(self): return self.action_space.sample() # Random action for now def act(self, action): return action
Set Up the Environment:
We’ll use OpenAI Gym’s CartPole environment as a simple test bed:
env = gym.make('CartPole-v1') agent = SimpleAgent(env.action_space)
Implement the Main Loop:
Now let’s create a loop where our agent interacts with the environment:
episodes = 10 for episode in range(episodes): observation = env.reset() done = False while not done: agent.perceive(observation) action = agent.decide() observation, reward, done, _ = env.step(agent.act(action)) print(f'Episode {episode + 1} finished')
This basic setup demonstrates the fundamental cycle of perception, decision-making, and action that defines an AI agent. Real-world AI agents are more complex, often incorporating advanced machine learning models, sophisticated sensor input processing, and complex decision-making algorithms.
Advancing Your AI Agent
To enhance your agent’s capabilities, consider these next steps:
- Implement a learning algorithm (e.g., Q-learning or policy gradients) to improve decision-making over time
- Enhance the perception module to process more complex sensory inputs
- Develop a more sophisticated action space to allow for nuanced interactions with the environment
- Integrate natural language processing for communication-based tasks
Remember, building an effective AI agent is an iterative process. Start simple, test thoroughly, and gradually increase complexity as you refine your understanding and skills in AI development. The future of AI agents is boundless. As we continue to push the boundaries of what’s possible, we’re not just creating smarter systems – we’re reshaping how we interact with technology and the world around us.
By following this guide and continuously expanding your knowledge, you’re well on your way to creating AI agents that can perceive, decide, and act in increasingly sophisticated ways. The journey of building AI agents is as much about understanding the underlying principles as it is about coding – embrace the learning process, and you’ll find yourself at the forefront of this exciting field.
Debugging and Optimizing AI Agents
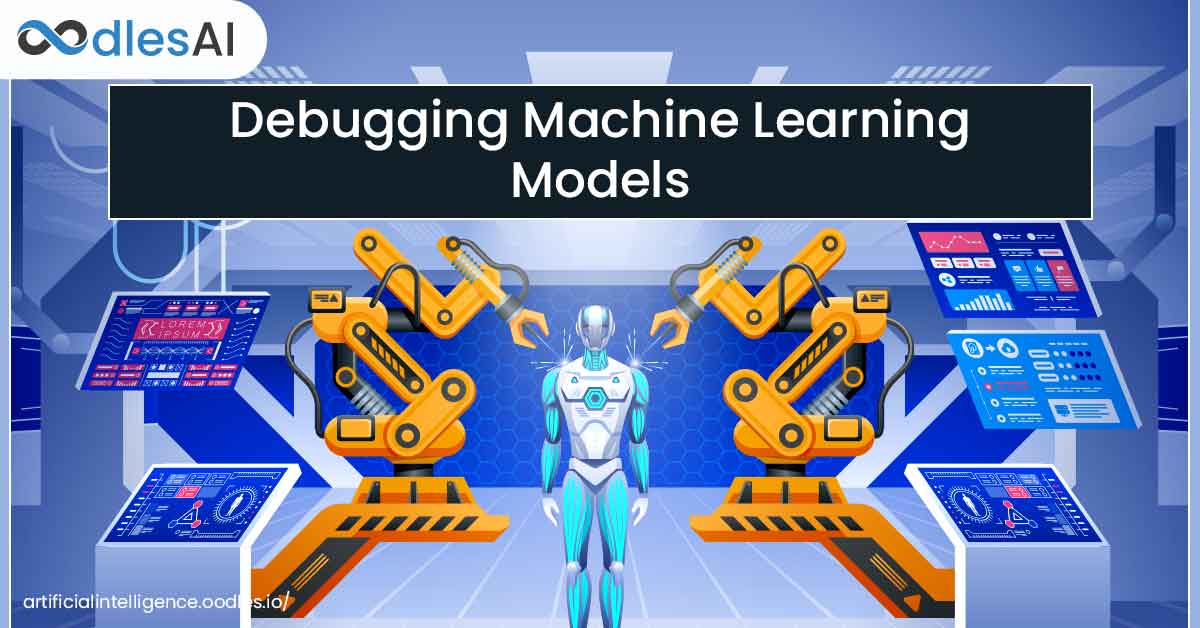
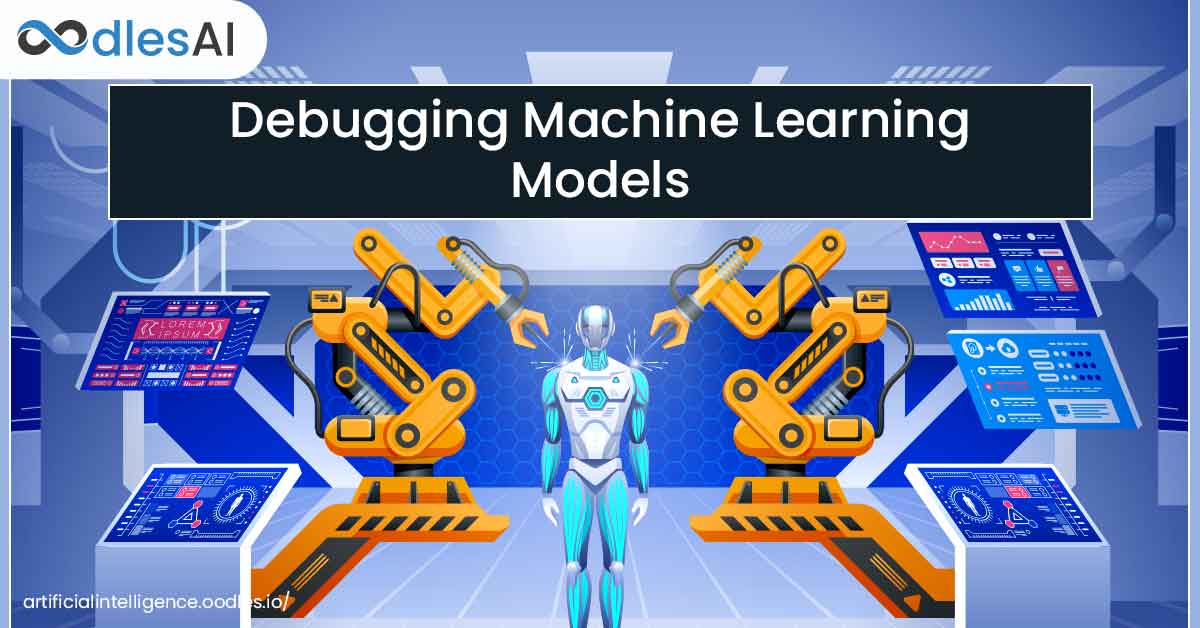
Debugging AI agents can feel like untangling a digital Gordian knot. These autonomous decision-makers often operate in ways that defy simple logic, making traditional debugging techniques feel inadequate. Yet, as AI becomes increasingly integrated into our systems, mastering the art of agent debugging is essential.
The challenge lies in the nature of AI agents. Unlike traditional software that follows a set of instructions, AI agents make decisions based on complex algorithms and vast datasets. This autonomy can lead to unexpected behaviors that are difficult to trace and correct.
Enter visual debugging environments—the game-changers in AI optimization. These tools offer developers a window into the ‘mind’ of an AI agent, allowing them to visualize decision pathways and identify potential pitfalls. It’s like having X-ray vision for code, revealing the inner workings of these digital decision-makers.
One platform making waves in this space is SmythOS. Their visual debugging tools demystify the behavior of AI agents, offering developers unprecedented insights into how these digital entities ‘think.’ By providing a clear visual representation of an agent’s decision-making process, SmythOS empowers developers to fine-tune their creations with precision.
But visual debugging is just the tip of the iceberg. Effective AI agent optimization involves a holistic approach that combines rigorous testing, continuous monitoring, and iterative refinement. It’s a process that demands patience, creativity, and a willingness to embrace the unexpected.
Debugging an AI agent is like teaching a child—you need to understand their perspective, guide them gently, and be prepared for a few surprises along the way. As we venture further into the age of artificial intelligence, the ability to effectively debug and optimize AI agents will become an increasingly valuable skill. It’s not just about fixing errors—it’s about shaping the future of how we interact with technology.
So roll up your sleeves, fire up that visual debugger, and get ready to embark on a journey of discovery in the fascinating world of AI agent optimization.
Empowering Developers with AI Agent Technology
The world of AI agents is rich with potential and complexity. From simple reflex agents to sophisticated learning models, artificial intelligence is evolving rapidly. For developers and technical leaders, the journey from concept to deployment can be both exhilarating and daunting. Success in AI agent development lies in understanding different agent types, mastering efficient development methodologies, and honing debugging skills. These elements form the foundation of creating AI solutions that are functional and transformative for businesses and end-users alike.
Enter SmythOS, a platform revolutionizing the AI development process. By offering a comprehensive suite of tools designed to streamline agent creation and deployment, SmythOS addresses many of the pain points developers face. Its visual workflow builder and reusable components significantly reduce the time and expertise required to bring AI agents to life, making the technology accessible to a broader range of professionals.
The practical applications of AI agents are virtually limitless. From enhancing customer service interactions to optimizing complex industrial processes, these digital helpers are poised to reshape how we work and interact with technology. For businesses looking to stay competitive, implementing AI agents is no longer a futuristic concept—it’s a present-day necessity.
As we look to the future, the importance of platforms like SmythOS becomes even clearer. By democratizing AI development, they’re fostering innovation and enabling businesses of all sizes to harness the power of artificial intelligence. The result? More efficient operations, enhanced decision-making capabilities, and the potential for groundbreaking new services and products.
The field of AI agent development is ripe with opportunity for those willing to dive in. With the right tools and knowledge at your disposal, you can turn complex AI concepts into practical, powerful solutions. Platforms like SmythOS are here to support you, making the path from idea to implementation smoother than ever before. The future of AI is not just coming—it’s here, and it’s waiting for innovators like you to shape it.
Last updated:
Disclaimer: The information presented in this article is for general informational purposes only and is provided as is. While we strive to keep the content up-to-date and accurate, we make no representations or warranties of any kind, express or implied, about the completeness, accuracy, reliability, suitability, or availability of the information contained in this article.
Any reliance you place on such information is strictly at your own risk. We reserve the right to make additions, deletions, or modifications to the contents of this article at any time without prior notice.
In no event will we be liable for any loss or damage including without limitation, indirect or consequential loss or damage, or any loss or damage whatsoever arising from loss of data, profits, or any other loss not specified herein arising out of, or in connection with, the use of this article.
Despite our best efforts, this article may contain oversights, errors, or omissions. If you notice any inaccuracies or have concerns about the content, please report them through our content feedback form. Your input helps us maintain the quality and reliability of our information.