How to Create an AI Agent with LangChain: A Step-by-Step Guide
Imagine having your own digital assistant that understands your needs and takes action to help you achieve them. That’s the power of AI agents, and today, you’ll learn how to create one yourself.
Building an AI agent is like assembling a sophisticated but manageable puzzle. Whether you want to automate repetitive tasks, enhance customer service, or develop innovative solutions, understanding the fundamentals of AI agent development opens up exciting possibilities. The best part? You don’t need to be an AI expert to get started.
In this comprehensive guide, you’ll find a step-by-step approach to creating your own AI agent. We’ll cover essential components like setting up your development environment, writing the core code, and integrating powerful libraries that give your agent its capabilities. You’ll learn how to handle real-world scenarios, manage your agent’s state, and implement error handling to ensure reliable performance.
Modern frameworks like LangChain have made AI agent development more accessible than ever. These tools provide the building blocks you need to create agents that can perceive their environment, make decisions, and take autonomous actions.
By the end of this journey, you’ll have the knowledge and practical skills to build an AI agent that can perform tasks independently, adapt to different situations, and help streamline your workflow. Let’s begin this exciting adventure into the world of AI agent development.
Understanding the Basics
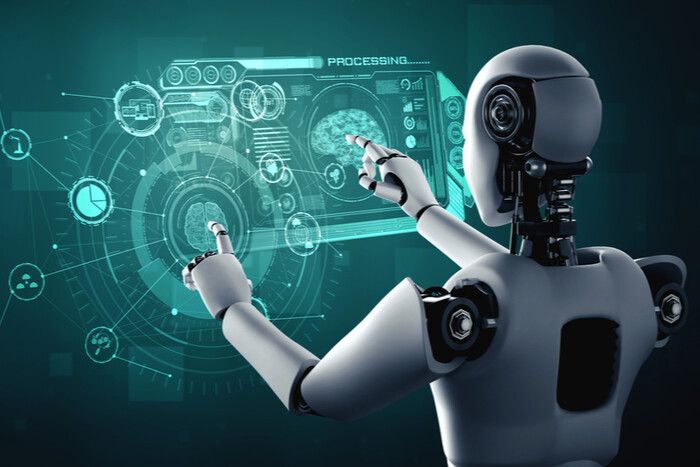
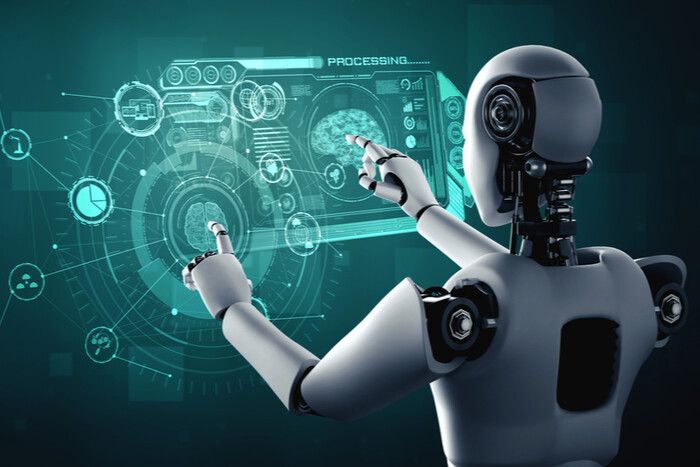
AI agents represent a fascinating breakthrough in artificial intelligence – autonomous systems that can perceive their surroundings, reason about what they observe, and take action to accomplish specific goals. Much like how humans process information and make decisions, these intelligent agents interact with their environment through a continuous cycle of observation and response.
The most straightforward type is the reactive agent, which operates on simple if-then rules based purely on current inputs. Think of a thermostat that turns the heat on when the temperature drops below a set point. While basic, reactive agents excel at handling clear-cut scenarios that don’t require memory or complex planning.
Moving up in sophistication, model-based agents maintain an internal representation of their environment. These agents can track changes over time and predict likely outcomes. For example, a self-driving car uses its model of the world to anticipate where other vehicles and pedestrians might move next.
Goal-based agents take things further by working toward specific objectives. Rather than just reacting or modeling, they evaluate different actions based on how well they achieve defined goals. A robot tasked with retrieving items in a warehouse exemplifies this type – it plans routes and sequences moves to efficiently collect the right products.
At the most advanced level, utility-based agents consider not just whether they can achieve goals, but optimize for the best possible outcomes. They weigh multiple factors like time, resources, and risk to maximize overall value. An AI trading system demonstrates this by balancing potential returns against investment risks when making decisions.
Each type of agent has its strengths, and understanding these foundational concepts is crucial for anyone looking to work with AI systems. The key is matching the right type of agent to your specific needs – whether that’s simple automation or complex decision-making requiring careful optimization.
Setting Up Your Development Environment
A well-configured development environment forms the foundation for building powerful AI agents. Follow these essential steps to set up your workspace properly and get started on the right path.
First, you need Python installed on your system (version 3.7 or higher is recommended). Python serves as the primary programming language for interacting with AI models and provides the necessary framework for your development work. To verify your Python installation, open your terminal and run python –version.
Next, install the required libraries using pip, Python’s package installer. The two core packages you need are OpenAI and LangChain. Execute these commands in your terminal:
pip install openai langchain
With the libraries installed, set up authentication for accessing OpenAI’s services. Create an account on the OpenAI platform and obtain your API key. This key acts as your secure credential for accessing OpenAI’s powerful language models. Store this key as an environment variable to maintain security:
export OPENAI_API_KEY=’your-api-key’
For Windows users, use the following command instead:
set OPENAI_API_KEY=your-api-key
Remember to replace ‘your-api-key’ with your actual OpenAI API key. Never share your API key or commit it directly in your code. Consider using environment files (.env) for managing sensitive credentials in your development projects.
Testing Your Setup
To ensure everything is working correctly, create a simple test script in Python. This will verify that your environment is properly configured and ready for development:
from langchain import OpenAI
from langchain.chains import LLMChainllm = OpenAI()
print(“Environment setup successful!”)Example verification code
If this code runs without errors, congratulations! Your development environment is now ready for building AI agents. Proper configuration is crucial for avoiding common issues and ensuring smooth development later on.
Coding Your AI Agent
Building an AI agent from scratch requires careful planning and a solid foundation of core functions. Essential components are needed to create an agent that can understand user inputs, generate meaningful responses, and execute commands with precision.
The first step involves setting up the agent’s basic architecture. We start by initializing the agent’s core functions for processing input and managing responses. This includes establishing a connection with a language model API and creating methods for handling user queries.
For response generation, your agent needs sophisticated natural language processing capabilities. Consider implementing a method similar to this:
def generate_response(self, prompt): client = OpenAI() response = client.chat.completions.create(model=’gpt-4′, messages=[{“role”: “user”, “content”: prompt}])
Hamza Farooq, Towards Data Science
The decision-making framework forms the heart of your AI agent. This involves creating conditional logic structures that evaluate user input and determine appropriate actions. Your agent should be able to parse commands, understand context, and choose the most relevant response or action based on predefined rules and learned patterns.
Executing commands requires security and validation. Implement strict input validation and establish clear boundaries for what commands your agent can execute. This prevents potential security risks while ensuring reliable performance. For instance, you might want to create a whitelist of allowed commands or implement rate limiting to prevent overuse.
Measure | Description |
---|---|
Input Validation | Ensures only valid data is accepted, preventing malicious code injection. |
Sanitization | Cleans user input by escaping special characters and applying filters. |
Parameterized Queries | Separates user input from SQL queries, preventing user input from being treated as part of the query. |
Prepared Statements | Pre-compiles SQL queries to enhance security. |
Least Privilege Principle | Grants users, applications, and systems only the essential level of access and permissions required for their tasks. |
Secure Network Configurations | Configures firewall rules and permissions to control permitted connections. |
Code Signing | Ensures that scripts and modules are signed to verify their authenticity. |
Execution Policies | Restricts execution of all PowerShell content to enhance security. |
Logging and Monitoring | Tracks error patterns and system performance over time for diagnosing issues and implementing preventive measures. |
Retry Mechanisms | Handles temporary failures in external service calls with exponential backoff. |
Remember to include error handling mechanisms throughout your code. AI agents often encounter unexpected inputs or scenarios, and robust error handling ensures graceful failure recovery rather than complete system breakdown. Include try-except blocks for critical operations and implement logging to track any issues that arise during execution.
Testing your agent’s capabilities should be an iterative process. Start with simple commands and gradually increase complexity as you verify each component works as intended. This methodical approach helps identify and fix issues early in the development process while ensuring your agent remains stable and reliable as it grows more sophisticated.
Integrating Essential Libraries
Building a robust AI agent requires carefully selected libraries that work together seamlessly. Two foundational libraries stand out for their powerful capabilities: OpenAI and LangChain. Here’s how to integrate these essential tools into your project.
OpenAI’s library serves as the cornerstone for advanced language processing capabilities. To get started, create an OpenAI account and generate an API key. Once you have your credentials, install the library using pip and set up your environment variables for secure access. LangChain acts as a sophisticated framework that simplifies complex interactions with language models. It provides the scaffolding needed to build applications that can understand context, manage conversations, and execute multi-step workflows. The library excels at streamlining development by handling various components of LLM interactions seamlessly. Combining these libraries provides a comprehensive toolkit that enables your agent to process natural language, generate responses, and maintain contextual awareness.
LangChain’s architecture complements OpenAI’s capabilities by providing structured ways to manage conversation memory, chain multiple operations, and customize model parameters. The integration of these libraries empowers you to develop various AI-driven solutions, from simple text generation to complex conversational agents and multi-step workflows. Gary Svenson, AI Developer
Setting Up Your Development Environment
Begin by installing the required packages through pip. Start with installing the LangChain framework and OpenAI libraries. This foundation ensures your agent can access both the core language model capabilities and the advanced features needed for complex tasks. Security should be a priority when handling API credentials. Implement environment variables to manage your OpenAI API key securely, preventing exposure in your codebase. This approach allows for easier deployment and better credential management across different environments.
Error handling becomes crucial when working with these integrations. Implement try-except blocks to gracefully handle API responses and potential exceptions. This ensures your agent remains stable and user-friendly, even when encountering unexpected situations. Consider caching mechanisms for improved performance. When building real-time applications, implementing response caching can significantly reduce latency and API costs while maintaining responsiveness. Finally, test your integrations thoroughly before deployment. Start with simple text generation tasks and gradually progress to more complex workflows. This incremental approach helps identify and resolve integration issues early in the development process.
State Management and Error Handling
Effective state management and error handling form the backbone of reliable AI agent systems. AI agents must gracefully handle unexpected situations while maintaining their operational state. Here’s how to implement these crucial capabilities to create more resilient and user-friendly AI systems.
Implementing Robust State Management
State management in AI agents involves tracking conversation context, user preferences, and operational parameters across multiple interactions. Think of it as the agent’s working memory—it needs to remember relevant information while forgetting what’s no longer needed.
A well-designed state management system should include mechanisms for storing both short-term conversational context and long-term user preferences. For example, in a customer service scenario, the agent should maintain context about the current issue while also remembering the customer’s previous interactions and preferences.
One effective approach is implementing a persistence layer using lightweight databases like SQLite. This enables agents to maintain state across sessions while providing ACID compliance for reliable transactions. The system should also include automated cleanup procedures to prevent unlimited growth of the state database.
Proper state management also requires careful handling of concurrent access in multi-threaded environments. Implementing thread-local storage and appropriate locking mechanisms helps prevent race conditions and data corruption when multiple instances of an agent are operating simultaneously.
Technique | Best For | Advantages | Disadvantages |
---|---|---|---|
Simple Service-Based State Management | Small to medium applications | Easy to implement, low complexity | Not suitable for complex state management |
Angular Signals | UI state that changes frequently | Fine-grained reactivity, better performance | Limited to Angular 16+ and specific use cases |
NgRx | Large and complex applications | Robust, scalable, unidirectional data flow | High complexity, steep learning curve |
Component State | Small to moderately complex applications | Simple and straightforward | Limited data sharing and async operations |
Services and RxJS | Medium to large-scale applications | Flexible, powerful for async data flows | Can become complex with large data streams |
Regular state checkpointing at meaningful decision points allows agents to resume operations seamlessly after interruptions. This is particularly important for long-running processes that may need to pause while waiting for external input or approval.
Graceful Error Handling Strategies
Research has shown that AI agents that openly acknowledge mistakes and handle them transparently are perceived as more intelligent and trustworthy by users.
Implementing comprehensive input validation serves as the first line of defense against errors. This includes checking for unexpected formats, empty values, and inappropriate content before processing begins. When errors do occur, the system should provide clear, user-friendly error messages that explain what went wrong without using technical jargon.
Graceful degradation is another crucial strategy—when faced with errors, agents should maintain functionality at a reduced level rather than failing completely. For instance, if a primary data source is unavailable, the agent can fall back to cached responses or simpler processing modes.
Establishing robust logging and monitoring systems helps track error patterns and system performance over time. This data proves invaluable for diagnosing issues and implementing preventive measures. Consider using structured logging with clearly defined error levels and contextual information.
Finally, implement retry mechanisms with exponential backoff for handling temporary failures in external service calls. This approach helps prevent system overload while maximizing the chances of successful recovery from transient errors.
Testing and Improving Your AI Agent
Think of your AI agent as a sophisticated digital employee who needs continuous training and feedback to excel. Regular testing is essential for building a reliable, high-performing AI system.
Start with pilot testing in a controlled environment. As industry experts recommend, this initial phase helps evaluate your agent’s effectiveness and identify potential issues before full deployment. Monitor how your agent handles different scenarios, edge cases, and unexpected inputs to ensure robust performance.
User feedback serves as your agent’s compass for improvement. Set up systematic ways to gather insights from actual users interacting with your agent. This could include direct feedback forms, monitoring user satisfaction scores, or analyzing interaction logs. Pay special attention to situations where users struggle or abandon their interactions, as these often reveal critical areas for enhancement.
When analyzing performance, focus on key metrics that matter. Track response accuracy, task completion rates, and user satisfaction scores.
Metric | Description |
---|---|
Accuracy | Reflects the AI agent’s ability to perform tasks with precision and correctness. |
Performance Speed | Measures how quickly an AI agent can complete tasks or process data. |
Reliability | Assesses the consistency and dependability of AI agents over time. |
Scalability | Refers to an AI agent’s ability to handle increased workloads without a significant drop in performance. |
Cost-Efficiency | Evaluates the financial effectiveness of deploying and maintaining AI agents relative to the benefits they provide. |
Integration Capability | Measures an AI agent’s ability to seamlessly connect with existing systems and processes within an enterprise. |
User Experience (UX) | Evaluates how users interact with and perceive an AI agent, including ease of use and satisfaction. |
Adaptability | Measures an AI agent’s ability to adjust to new data, changing conditions, and evolving requirements. |
Security | Assesses how well an AI agent protects data and operates within secure parameters. |
Compliance | Measures an AI agent’s adherence to relevant laws, regulations, and industry standards. |
Data Handling and Management | Evaluates an AI agent’s capability to efficiently process, store, and utilize data. |
Customer Support | Evaluates how effectively an AI agent assists users and resolves issues related to its functionalities. |
Innovation | Measures the extent to which an AI agent introduces new features, capabilities, or improvements. |
Training and Maintenance | Evaluates the resources and efforts required to keep an AI agent functioning optimally over time. |
User Adoption | Measures how effectively users embrace and utilize an AI agent within the enterprise. |
But don’t just collect data—use it to make informed decisions about improvements. If users consistently struggle with certain queries, that’s a clear signal to enhance your agent’s understanding in those areas.
Remember that improvement is an iterative process. Each round of testing and feedback should lead to specific, actionable updates to your agent’s capabilities. Whether it’s fine-tuning response patterns, expanding knowledge bases, or adjusting interaction flows, make changes methodically and measure their impact.
Most importantly, maintain a balance between automation and human oversight. While AI agents can handle many tasks independently, regular human review ensures they stay aligned with your goals and maintain high-quality standards. This hybrid approach helps catch potential issues early and guides continuous improvement efforts.
The age of AI agents is upon us, and those who embrace continuous testing and improvement will be the architects of tomorrow’s success stories.
Sangita Machhi, AI Implementation Specialist
Consider implementing automated testing routines that can run 24/7, checking your agent’s responses against predefined scenarios. This continuous testing approach helps maintain consistency and catches any performance degradation early. When issues are identified, address them promptly to prevent them from affecting user experience.
Using SmythOS for AI Agent Development
SmythOS simplifies the creation of AI agents for various purposes. There are two main types: brand agents that interact with customers and process agents that assist with internal tasks. The advantage of SmythOS is its user-friendly interface, which doesn’t require coding expertise.
A standout feature of SmythOS is its visual workflow builder. This tool allows users to design AI agents by dragging and dropping different components, similar to assembling a puzzle but creating a functional AI helper.
SmythOS also offers reusable components, which act as building blocks for different projects. This feature significantly reduces development time and accelerates the creation of AI agents.
Companies can quickly develop AI agents to handle tasks such as answering customer inquiries or organizing work processes using SmythOS. Its intuitive tools enable broader participation in AI development within a company, extending beyond tech experts.
SmythOS makes developing intelligent agents easier, whether for improving customer interactions or streamlining work processes, without the need for complex coding.
Conclusion and Future Directions
The journey of AI agent development represents a significant shift in how we approach automation and intelligence in computing systems. From establishing foundational components to implementing sophisticated learning mechanisms, each step in creating an AI agent demands careful consideration and expertise. This process encompasses not just coding, but a comprehensive understanding of AI architectures, data handling, and system integration.
Research from MIT suggests that future AI agents will demonstrate unprecedented levels of autonomy and adaptability, fundamentally changing our interactions with automated systems. These advancements drive innovations in customer service and complex decision-making processes. The integration of emerging technologies like quantum computing and advanced neural networks promises to push the boundaries of AI capabilities. We’re moving beyond simple task automation toward systems capable of nuanced reasoning and sophisticated problem-solving. This evolution will likely transform industries, creating new opportunities while challenging traditional AI development approaches.
Testing and refinement remain crucial as these systems grow more complex. Continuous evaluation ensures AI agents not only meet current requirements but can adapt to future challenges. Robust testing frameworks and iterative improvement cycles will become increasingly important as AI agents take on more critical roles across various sectors. AI agent development isn’t just about creating more powerful systems—it’s about building intelligent solutions that can effectively collaborate with humans while maintaining reliability and ethical standards. The future of AI agents lies in their technical capabilities and their ability to seamlessly integrate into our daily lives and work environments.
Last updated:
Disclaimer: The information presented in this article is for general informational purposes only and is provided as is. While we strive to keep the content up-to-date and accurate, we make no representations or warranties of any kind, express or implied, about the completeness, accuracy, reliability, suitability, or availability of the information contained in this article.
Any reliance you place on such information is strictly at your own risk. We reserve the right to make additions, deletions, or modifications to the contents of this article at any time without prior notice.
In no event will we be liable for any loss or damage including without limitation, indirect or consequential loss or damage, or any loss or damage whatsoever arising from loss of data, profits, or any other loss not specified herein arising out of, or in connection with, the use of this article.
Despite our best efforts, this article may contain oversights, errors, or omissions. If you notice any inaccuracies or have concerns about the content, please report them through our content feedback form. Your input helps us maintain the quality and reliability of our information.